Typescript SDK
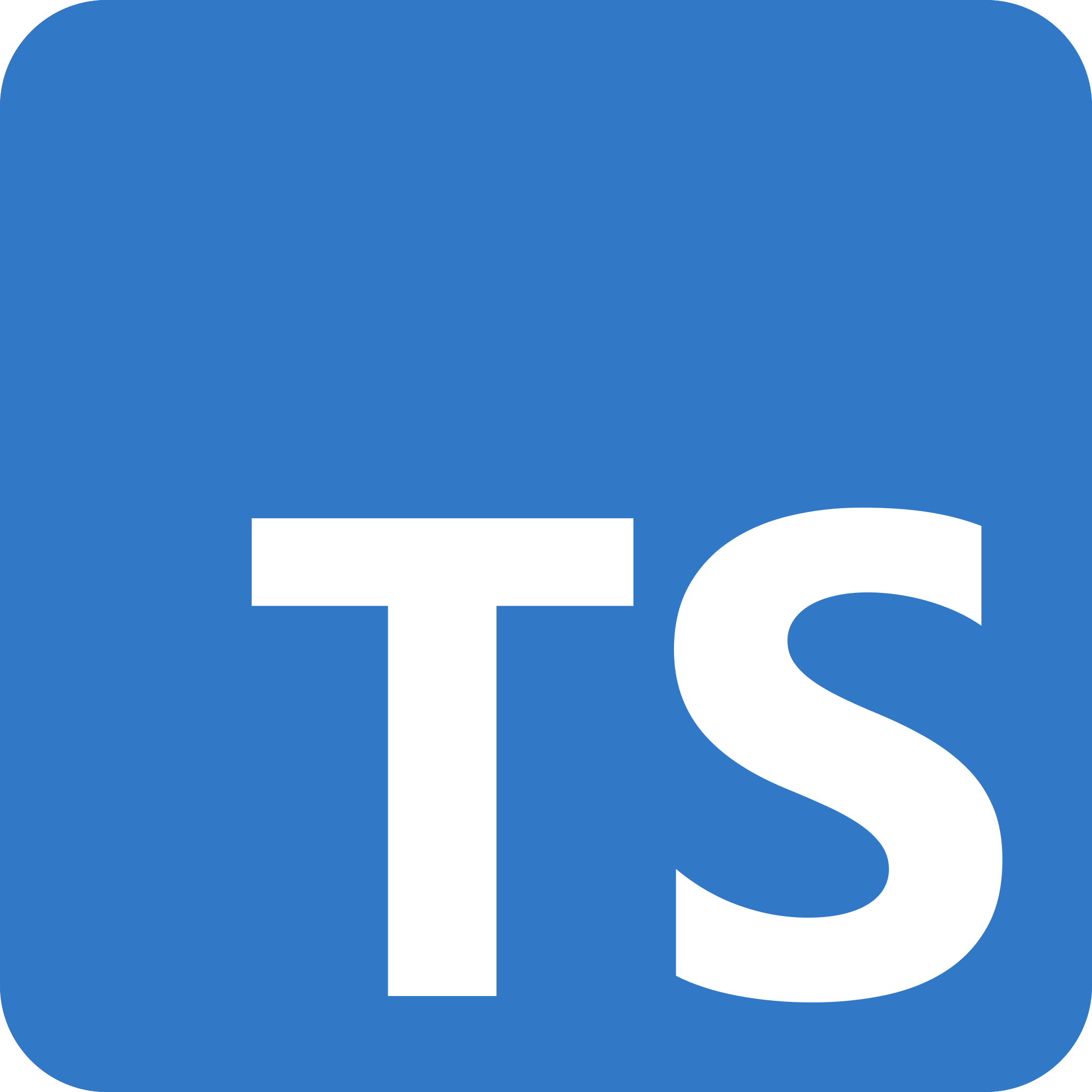
We provide a Typescript SDK for the Node developers out there. Our package needle is available on NPM JS and can be installed like so:
npm install @needle-ai/needle
or using the package manager of our choice:
bun install @needle-ai/needle
Quickstart
Usage is very simple and similar to the Python SDK. Get your API key in Needle Settings. Note that your key will be valid until you revoke it.
Set the following env variable before you run your code:
export NEEDLE_API_KEY=<your-api-key>
Needle
reads the API key from the environment by default. If you like to override this behaviour you can pass it in as a parameter.
Retrieve context from Needle
import { Needle } from "@needle-ai/needle/v1";
const needle = new Needle();
const prompt =
"What techniques moved into adopt in this volume of technology radar?";
const results = await needle.collections.search({
collection_id: "<collection-id>",
text: prompt,
});
Complete your RAG pipeline
To compose a human friendly answer use an LLM provider of your choice. For the demo, we use OpenAI in this example:
import OpenAI from "openai";
import { type ChatCompletionMessageParam } from "openai/src/resources/index.js";
const openai = new OpenAI();
const messages: ChatCompletionMessageParam[] = [
{
role: "system",
content: `
You are a helpful assistant. Answer the question based on the context.
Context:
${results.map((r) => r.content).join("\n")}
`,
},
{ role: "user", content: prompt },
];
const completion = await openai.chat.completions.create({
model: "gpt-4o-mini",
messages,
});
const answer = completion.choices[0].message.content;
console.log(answer);
As you see, you can quickly implement this example RAG pipeline using Needle and OpenAI. Needle API helps you with hassle-free contextualization however does not limit you to a certain RAG technique. You can furthermore add Needle search as a tool to your agentic application to allow LLMs trigger search autonomously.
Additional Resources
See our API Reference for the extensive list of supported functionality.
Support
If you have questions you can contact us in our Discord channel.
License
needle-typescript
is distributed under the terms of the MIT license.