Build AI Agents with Haystack + Needle
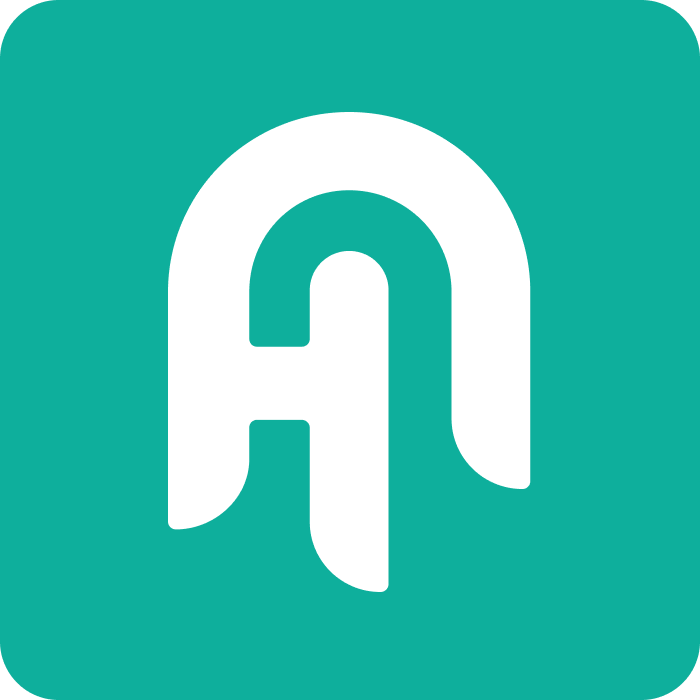
The Needle Haystack integration provides powerful RAG components for Haystack projects, including NeedleDocumentStore
and NeedleEmbeddingRetriever
. These components allow you to seamlessly integrate Needle's advanced search capabilities into your Haystack pipelines.
Installation
Install the package via pip:
pip install needle-haystack-ai
API Keys
To use the Needle Haystack integration, you'll need to set up your environment variables:
NEEDLE_API_KEY
- Get your API key from Developer settingsOPENAI_API_KEY
- Required if using OpenAI in your pipeline
Components
NeedleDocumentStore
The NeedleDocumentStore
component provides a connection to your Needle collections. In Needle, document stores are called collections. For detailed information about collections, see our Collections documentation.
NeedleEmbeddingRetriever
The NeedleEmbeddingRetriever
component enables semantic search over your documents using Needle's advanced retrieval capabilities.
Example Pipeline
Here's how to build a RAG pipeline using Needle components with Haystack:
from needle_haystack import NeedleDocumentStore, NeedleEmbeddingRetriever
from haystack import Pipeline
from haystack.components.generators import OpenAIGenerator
from haystack.components.builders import PromptBuilder
# Initialize Needle components
document_store = NeedleDocumentStore(collection_id="<your-collection-id>")
retriever = NeedleEmbeddingRetriever(document_store=document_store)
# Create prompt template
prompt_template = """
Given the following retrieved documents, generate a concise and informative answer to the query:
Query: {{query}}
Documents:
{% for doc in documents %}
{{ doc.content }}
{% endfor %}
Answer:
"""
# Set up pipeline components
prompt_builder = PromptBuilder(template=prompt_template)
llm = OpenAIGenerator()
# Create and configure pipeline
pipeline = Pipeline()
pipeline.add_component("retriever", retriever)
pipeline.add_component("prompt_builder", prompt_builder)
pipeline.add_component("llm", llm)
# Connect components
pipeline.connect("retriever", "prompt_builder.documents")
pipeline.connect("prompt_builder", "llm")
# Run the pipeline
prompt = "What is the topic of the news?"
result = pipeline.run({
"retriever": {"text": prompt},
"prompt_builder": {"query": prompt}
})
# Get the response
print(result['llm']['replies'][0])
Support
If you have questions or need assistance:
- Join our Discord community
- Check our API Reference
- Visit our GitHub repository
License
The Needle Haystack integration is distributed under the terms of the MIT license.