Building a RAG Chatbot Made Simple with Needle TypeScript SDK
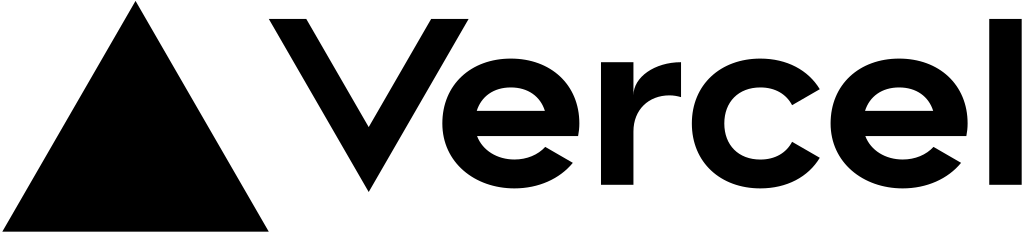
In the world of AI development, efficiency and simplicity are crucial. Developers often seek solutions that not only enhance functionality but also reduce complexity and save time. Retrieval-Augmented Generation (RAG) chatbots are a prime example where these attributes are needed. While Vercel AI SDK provides a robust toolkit for building AI applications, the Needle TypeScript SDK offers a faster and more straightforward alternative for creating RAG pipelines.
What is RAG and Why Does it Matter?
Retrieval-Augmented Generation (RAG) enhances the capabilities of Large Language Models (LLMs) by integrating external knowledge bases. This approach is invaluable for providing contextually rich and accurate responses, especially when dealing with proprietary or up-to-date information. By fetching relevant data and using it as context for the LLM, RAG enables applications to generate more accurate and reliable responses.
For example:
Input: What is my favorite food?
Model Response (without RAG): I don't have access to personal information.
With RAG Context:
User loves Avocado Egg Toast.
Model Response: Your favorite food is Avocado Egg Toast!
The Challenge with Vercel AI SDK
The Vercel AI SDK offers a comprehensive framework for building RAG applications. However, its complexity can be daunting, involving:
- Extensive Setup: Initializing Next.js, configuring AI SDK, and integrating OpenAI
- Embedding Management: Setting up PostgreSQL with pgvector, chunking data, and embedding logic
- Complex Tool Definition: Defining tools and managing multi-step operations with intricate configurations
- API Overhead: Creating endpoints, managing server-side logic, and handling responses
While thorough, these steps require significant time and effort, making the development process cumbersome.
Enter Needle: A Simpler Path to RAG Chatbots
Needle TypeScript SDK takes the hassle out of building RAG chatbots. It provides a streamlined, out-of-the-box solution for managing RAG pipelines. With minimal setup and intuitive APIs, you can achieve what the Vercel SDK requires in a fraction of the time.
Step-by-Step Guide to Building a RAG Chatbot with Needle
Here's how you can quickly build a RAG chatbot using Needle SDK:
1. Install and Set Up Needle SDK
First, install the Needle SDK:
bun install @needle-ai/needle
export NEEDLE_API_KEY=<your-api-key>
2. Define a Retrieval Tool
The Needle SDK simplifies the process of defining tools for your chatbot. Here's an example of a makeSearchCollectionTool function that retrieves information from a knowledge base:
import { Needle } from "@needle-ai/needle/v1";
import { tool } from 'ai';
import { z } from 'zod';
const ndl = new Needle();
function makeSearchCollectionTool(collectionId: string) {
return tool({
description: `Retrieve information from a knowledge base.`,
parameters: z.object({
question: z.string().describe("User's query."),
}),
execute: async ({ question }) => (
ndl.collections.search({
collection_id: collectionId,
text: question,
})
),
});
}
This tool enables the chatbot to query Needle's managed RAG pipeline effortlessly.
3. Create the Chatbot API
With Needle, setting up the chatbot's backend is straightforward:
import { generateText, tool } from 'ai';
const result = await generateText({
model: 'gpt-4o-mini',
tools: {
searchCollection: makeSearchCollectionTool("<collection-id>")
},
prompt: 'Explain the latest advancements in AI.',
maxSteps: 5, // Allow the model to retrieve data up to 5 times.
});
console.log(result.text);
This code configures the chatbot to use Needle for retrieving context during its interactions.
4. Run Your Chatbot
Deploy the backend and connect it to a simple frontend using the useChat hook (if needed). The chatbot is now ready to answer queries with accurate, context-aware responses.
Why Choose Needle Over Manual RAG Setup?
1. Reduced Complexity
Needle abstracts embedding management, vector storage, and server configurations. Developers can focus on core features without diving into low-level details.
2. Faster Development
What takes dozens of steps in the Vercel SDK can be accomplished with a few lines of code in Needle.
3. Security-First Design
Needle ensures secure authentication using API keys externally, while internally it leverages session IDs.
4. Out-of-the-Box Functionality
Needle's managed RAG pipelines handle data retrieval seamlessly, eliminating the need to set up databases or configure embeddings.
Support
Need help? We're here: